WinBox.js 是一个现代的 HTML5 web 窗口管理器。轻量级、性能出色、无依赖、完全可定制、免费且开源!
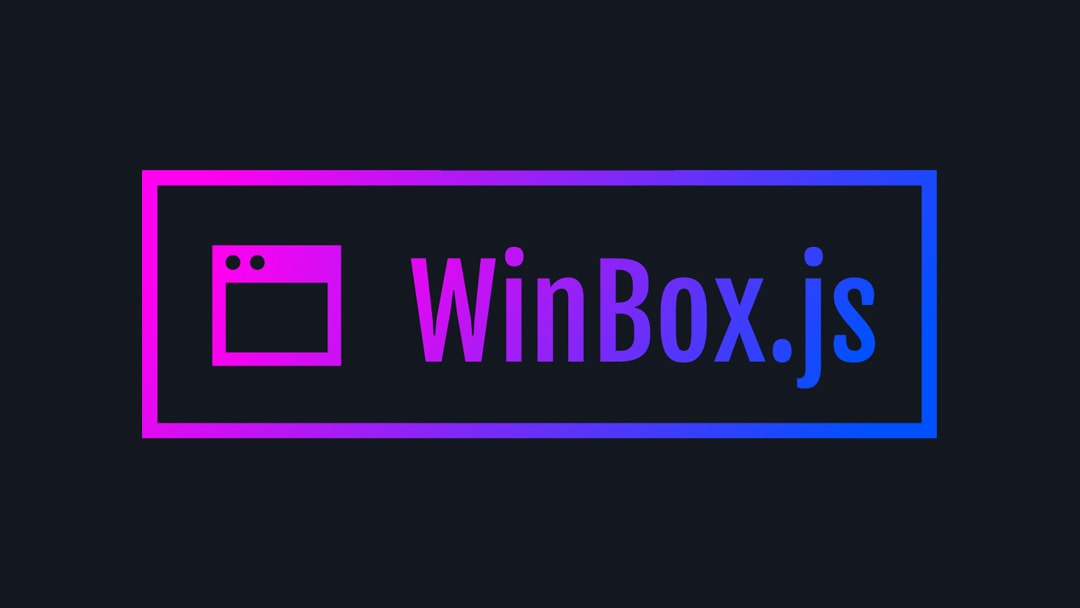
引入
使用NPM
npm install winbox
使用捆绑版本
捆绑版本包括所有 js、css、html 和 base64 图标图片。
<html>
<head>
<script src="winbox.bundle.js"></script>
</head>
<body></body>
</html>
使用非捆绑版本
非捆绑版本需要分别加载 js 和 css(css也包含base64图标)。
<html>
<head>
<link rel="stylesheet" href="winbox.min.css">
<script src="winbox.min.js"></script>
</head>
<body></body>
</html>
预加载库/异步加载(推荐)
根据你的代码,你可能需要以正确的顺序加载它们。
<html>
<head>
<title></title>
<link rel="preload" href="winbox.bundle.js" as="script">
</head>
<body>
<!--
HTML CONTENT
-->
<!-- BOTTOM OF BODY -->
<script src="winbox.bundle.js" defer></script>
<!-- YOUR SCRIPT -->
<script src="my-script.js" defer></script>
</body>
</html>
ES6 模块
ES6 模块位于 src/js/ 中。 你需要显式加载样式表文件(包括 base64 格式的图标)。
<head>
<link rel="stylesheet" href="dist/css/winbox.min.css">
</head>
<script type="module">
import WinBox from "./src/js/winbox.js";
</script>
你还可以通过 CDN 加载模块
<script type="module">
import WinBox from "https://unpkg.com/[email protected]/src/js/winbox.js";
</script>
选项
选项 | 值 | 说明 |
id | number | string | 为窗口设置一个唯一的 id。 用于在 css 中自定义样式,通过上下文查询元素或仅用于标识相应的窗口实例。 如果未设置 ID,它将自动为你创建一个。 |
index | number | 将窗口的初始 z-index 设置为此值(未聚焦/聚焦时可以自动增加)。 |
title | string | 窗口标题。 |
mount | HTMLElement | 将元素(小部件、模板等)安装到窗口主体。 |
html | string | 设置窗口主体的 innerHTML。 |
url | string | 在窗口内打开 URL(通过 iframe 加载)。 |
width height |
number | string | 设置窗口的初始宽度/高度(支持单位“px”和“%”)。 |
minwidth minheight |
number | string | 设置窗口的最小宽度/高度(支持单位“px”和“%”)。 |
x y |
number | string | 设置窗口的初始位置(支持:x轴为“右”,y轴为“底部”,两者都有为“中心”,两者的单位为“px”和“%”)。 |
max | boolean | 创建时自动将窗口切换到最大化状态。 |
top right bottom left |
number | string | 设置或限制窗口可用区域的视口(支持单位“px”和“%”)。 |
background | string | 设置窗口的背景(支持所有CSS样式,例如颜色、透明度、hsl、渐变、背景图像) |
border | number | 设置窗口的边框宽度(支持所有 css 单位,如 px、%、em、rem、vh、vmax)。 |
class | string | 向窗口添加一个或多个类名(多个类名作为数组或用空格分隔,例如“class-a class-b”)。 用于在 css 中定义自定义样式,按上下文(也在 CSS 中)查询元素或仅标记相应的窗口实例。
WinBox 提供了一些有用的内置控件类来轻松设置自定义配置。 |
modal | boolean | 将窗口显示为模态窗。 |
onmove | function(x, y) | 窗口移动时触发的回调。 回调函数中的关键字 this 是指对应的 WinBox 实例。 |
onresize | function(width, height) | 当窗口调整大小时触发回调。 回调函数中的关键字 this 是指对应的 WinBox 实例。 |
onclose | function(force) | 窗口关闭时触发的回调。 回调函数中的关键字 this 是指对应的 WinBox 实例。 注意:事件 ‘onclose’ 将在关闭之前触发,并在应用回调并返回真值时停止关闭。 |
onfocus onblur |
function() | 对多个事件的回调。 回调函数中的关键字 this 是指对应的 WinBox 实例。 |
splitscreen | boolean | 启用分屏功能,通过在窗口旁边拖动来并排查看两个窗口 |
创建和设置窗口
基本窗口
当没有指定 root
时,窗口将附加到 document.body
。
new WinBox("Window Title");
或者:
WinBox.new("Window Title");
或者:
new WinBox({
title: "Window Title"
});
或者:
var winbox = WinBox();
winbox.setTitle("Window Title");
定制 root
root
是窗口将附加到的文档中的唯一元素。 在大多数情况下,这通常是作为默认 root
的 document.body
。 同时多个 root
只是部分支持(它们实际上共享相同的视口)。
new WinBox("Window Title", {
root: document.body
});
定制颜色
支持样式属性“background
”也支持所有 CSS 样式,例如 颜色、透明度、hsl、渐变、背景图像。
new WinBox("Custom Color", {
background: "#ff005d"
});
或者:
var winbox = new WinBox("Custom Color");
winbox.setBackground("#ff005d");
定制边框
支持所有单位。
new WinBox({
title: "Custom Border",
border: "1em"
});
你还可以定义多个边框值(顺序为:上、右、下、左):
new WinBox({
title: "Custom Border",
border: "0 1em 15px 1em"
});
定制视口
定义窗口可以移动或可以调整大小的可用区域(相对于文档)(支持单位“px”和“%”)。
new WinBox("Custom Viewport", {
top: "50px",
right: "5%",
bottom: 50,
left: "5%"
});
或者(只支持数字):
var winbox = new WinBox("Custom Viewport");
winbox.top = 50;
winbox.right = 200;
winbox.bottom = 0;
winbox.left = 200
定制位置/尺寸
支持 x 轴“右”,y 轴“底部”,两者都有为“中心”,单位“px”和“%”也都支持。
new WinBox("Custom Viewport", {
x: "center",
y: "center",
width: "50%",
height: "50%"
});
new WinBox("Custom Viewport", {
x: "right",
y: "bottom",
width: "50%",
height: "50%"
});
或者(也支持与上述相同的单位):
var winbox = new WinBox("Custom Viewport");
winbox.resize("50%", "50%")
.move("center", "center");
或者(只支持数字):
var winbox = new WinBox("Custom Viewport");
winbox.width = 200;
winbox.height = 200;
winbox.resize();
winbox.x = 100;
winbox.y = 100;
winbox.move();
模态窗
new WinBox({
title: "Modal Window",
modal: true
});
主题
你可以在这里找到所有主题。
加载相应的 css 文件(或使用打包器),例如:
<head>
<link rel="stylesheet" href="dist/css/winbox.min.css">
<link rel="stylesheet" href="dist/css/themes/modern.min.css">
<script src="dist/js/winbox.min.js"></script>
</head>
只需将主题名称添加为一个类:
var winbox = new WinBox({
title: "Theme: Modern",
class: "modern"
});
或者:
var winbox = new WinBox("Theme: Modern");
winbox.addClass("modern");
你可以在窗口的生命周期内更改主题。
窗口管理器内容
设置 innerHTML
不要忘记清理作为 html 一部分的任何用户输入,因为这可能导致意外的 XSS!
new WinBox("Set innerHTML", {
html: "<h1>Lorem Ipsum</h1>"
});
或者:
var winbox = new WinBox("Set innerHTML");
winbox.body.innerHTML = "<h1>Lorem Ipsum</h1>";
挂载 DOM(克隆)
通过克隆,你可以轻松地并行创建相同内容的多个窗口实例。
<div id="content">
<h1>Lorem Ipsum</h1>
<p>Lorem ipsum [...]</p>
</div>
var node = document.getElementById("content");
new WinBox("Mount DOM", {
mount: node.cloneNode(true)
});
或者:
var node = document.getElementById("content");
var winbox = new WinBox("Mount DOM");
winbox.mount(node.cloneNode(true));
更多详细英文文档请参照WinBox in Github。
2021-06-30