Rythm.js 是一个非常有意思的 JavaScript 库,它可以使页面中的元素跟随音乐的节奏跳动,包含多种动效,或许你可以用它来创建一些有趣的东西。
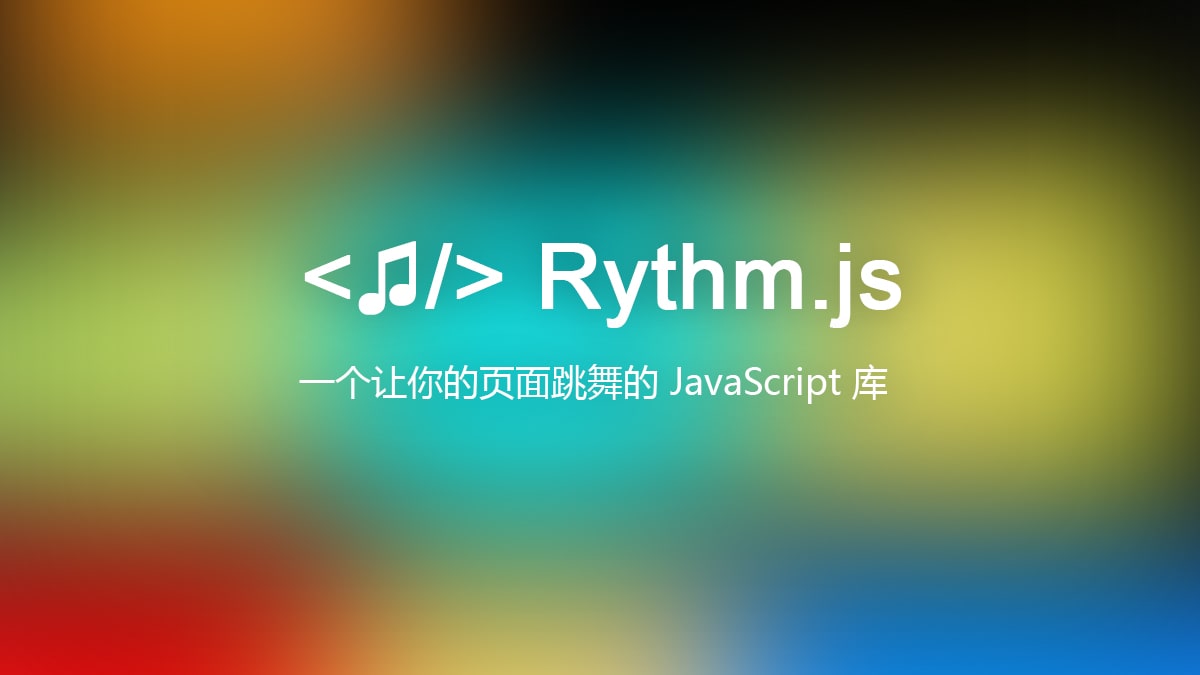
特色
- 你的 HTML 元素可以使用任何可用的动效类型。
- 你可以使用自定义函数来构建你自己的动效。
快速开始
使用 npm:
npm install rythm.js
使用 CDN:
https://unpkg.com/rythm.js/
https://cdnjs.cloudflare.com/ajax/libs/rythm.js/2.2.5/rythm.min.js
引入文件
将 rythm.js 导入到页面:
<script type="text/javascript" src="/path/to/rythm.min.js"></script>
添加预置的动效 css 类为元素附加动效:
<div class="rythm-bass"></div>
创建一个 rythm
对象并为其提供音频 URL,然后使用 start
函数:
var rythm = new Rythm()
rythm.setMusic('path/to/sample.mp3')
rythm.start()
ES6 module
import Rythm from 'rythm.js'
const rythm = new Rythm()
rythm.setMusic('path/to/sample.mp3')
rythm.start()
API
Rythm 对象
const rythm = new Rythm()
/* 起始 scale 是元素采用的最小 scale (Scale ratio is startingScale + (pulseRatio * currentPulse)).
* Value in percentage between 0 and 1
* Default: 0.75
*/
rythm.startingScale = value
/* 脉冲比是元素将采用的最大比例 (Scale ratio is startingScale + (pulseRatio * currentPulse)).
* Value in percentage between 0 and 1
* Default: 0.30
*/
rythm.pulseRatio = value
/* 最大历史值表示将被存储以评估当前脉冲的传递值的数量。
* Int value, minimum 1
* Default: 100
*/
rythm.maxValueHistory = value
/* 设置音乐
* @audioUrl: '../example/mysong.mp3'
*/
rythm.setMusic(audioUrl)
/* Used to collaborate with other players library.
* You can connect Rythm to an audioElement, and then control the audio with your other player
*/
rythm.connectExternalAudioElement(audioElement)
/* 调整音频增益
* @value: Number
*/
rythm.setGain(value)
/* 添加你自己的 rythm-class
* @elementClass: Class that you want to link your rythm to
* @danceType: Use any of the built-in effect or give your own function
* @startValue: The starting frequency of your rythm
* @nbValue: The number of frequency of your rythm
* 1024 Frequencies, your rythm will react to the average of your selected frequencies.
* Examples: bass 0-10 ; medium 150-40 ; high 500-100
*/
rythm.addRythm(elementClass, danceType, startValue, nbValue)
/* Plug your computer microphone to rythm.js.
* This function returns a Promise object that is resolved when the microphone is up.
* Require your website to be run in HTTPS
*/
rythm.plugMicrophone().then(function(){...})
// 开始
rythm.start()
/* 停止
* @freeze: Set this to true if you want to prevent the elements to reset to their initial position
*/
rythm.stop(freeze)
具有“脉冲”效果的内置类
- rythm-bass
- rythm-medium
- rythm-high
自定义类
你可以使用 addRythm
函数自定义类。以下是基础类的创建方式:
addRythm('rythm-bass', 'pulse', 0, 10)
addRythm('rythm-medium', 'pulse', 150, 40)
addRythm('rythm-high', 'pulse', 500, 100)
自定义 dance 类型
/* 自定义函数签名是
* @elem: 要应用效果的 HTML 元素目标
* @value: 当前脉冲比(0到1之间的百分比)
* @options: The option object user can give as last argument of addRythm function
*/
const pulse = (elem, value, options = {}) => {
const max = options.max || 1.25
const min = options.min || 0.75
const scale = (max - min) * value
elem.style.transform = `scale(${min + scale})`
}
/* 复位函数签名是
* @elem: The element to reset
*/
const resetPulse = elem => {
elem.style.transform = ''
}
addRythm('my-css-class', { dance: pulse, reset: resetPulse }, 150, 40)
2021-07-07